Tags
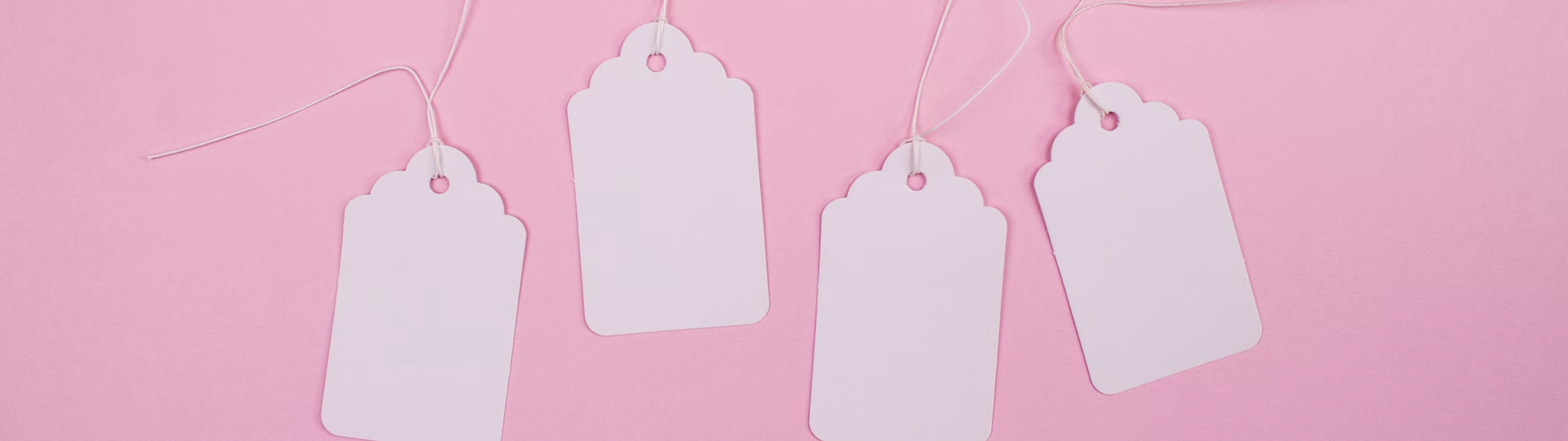
ZML offers a multitude of tags which are keywords defining how the platform will format and display the content. Let’s have a look at the tags ZML come with:
Comment
The comment
tag allows you to leave un-rendered code in the content. Any text within the opening and closing comment
blocks will not be output, and any ZML code within will not be executed.
Input
|
Output
|
Control Flow
Control flow tags can change the information ZML shows using logic:
If
Executes a block of code only if a certain condition is true
.
Input
|
Output
|
Unless
The opposite of if
– executes a block of code only if a certain condition is not met.
Input
|
Because first_name in our example is equal to Ryan, the output returns the first name. If our first_name was Tom, the output would return an empty value.
Output
|
Elsif / Else
Adds more conditions within an if
or unless
block. Note the missing 'e' in elsif
; it is intentional.
Input
|
Case / When
Creates a switch statement to compare a variable with different values. case
initializes the switch statement, and when
compares its values.
Input
|
Output
|
Iteration
Iteration tags run blocks of code repeatedly:
For
Repeatedly executes a block of code. For a full list of attributes available within a for
loop, see forloop (object).
Input
|
Output
|
Break
Causes the loop to stop iterating when it encounters the break
tag.
Input
|
Output
|
Continue
Causes the loop to skip the current iteration when it encounters the continue
tag.
Input
|
Output
|
For (Parameters)
Limit
Limits the loop to the specified number of iterations.
Input
|
Output
|
Offset
Begins the loop at the specified index.
Input
|
Output
|
Range
Defines a range of numbers to loop through. The range can be defined by both literal and variable numbers.
Input
|
Output
|
Reversed
Reverses the order of the loop. Note that the flag’s spelling is different from the filter reverse
.
Input
|
Output
|
Cycle
Loops through a group of strings and outputs them in the order that they were passed as parameters. Each time cycle
is called, the next string that was passed as a parameter is output.
cycle
must be used within a for loop block.
Input
|
Output
|
Uses for cycle
include:
Alternating between odd/even rows
applying a unique class to the last product thumbnail in a row
Cycle (Parameters)
cycle
accepts a parameter called cycle group
in cases where you need multiple cycle
blocks in one template. If no name is supplied for the cycle group, then it is assumed that multiple calls with the same parameters are one group. More information on cycle groups can be found here.
Tablerow
See here for more information on creating tables with ZML.
Raw
Raw temporarily disables tag processing. This is useful for generating content (eg, Mustache, Handlebars) that uses conflicting syntax but may not have much usefulness within content end users will see.
Input
|
Output
|
Variable
Variable tags create new ZML variables.
Assign
Creates a new variable.
Input
|
Output
|
Wrap a variable in quotations "
to save it as a string.
Input
|
Output
|
Capture
Captures the string inside of the opening and closing tags and assigns it to a variable. Variables created through {% capture %}
are strings.
Input
|
Output
|
Using capture
, you can create complex strings using other variables created with assign
.
Input
|
Output
|
Increment
Creates a new number variable, and increases its value by one every time it is called. The initial value is 0.
Input
|
Output
|
Variables created through the increment
tag are independent from variables created through assign
or capture
.
In the example below, a variable named “var” is created through assign
. The increment
tag is then used several times on a variable with the same name. Note that the increment
tag does not affect the value of “var” that was created through assign
.
Input
|
Output
|
Decrement
Creates a new number variable, and decreases its value by one every time it is called. The initial value is -1. Like increment, variables declared inside decrement
are independent of variables created through assign
or capture
.
Set Global Variables
You can use the global
tag to set a global variable to populate information in the Subject Line and Preheader from properties and resources defined in the campaign body.
For Example,
|
List of Segments
Getting a list of Segments in the content enables you to serve dynamic content based on a user's segment membership. Use {% segments segment_names %}
in content to fetch all segments that the targeted user is part of. This returns the data structured as follows:
|
Additionally, a map
filter can be used to get a simple array in ZML for ease of operating on this data.
|