Filters
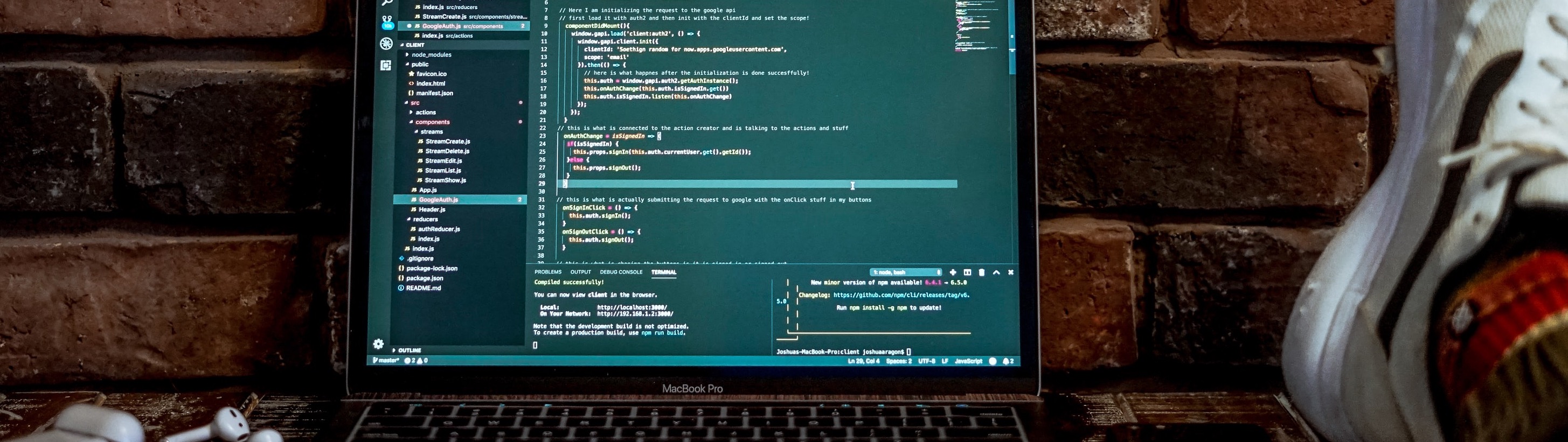
Filters are used when the output of a ZML object needs to be modified, be it in the case of text change, performing math functions, for encoding and decoding of strings, and more. They are used within an output and are separated by a |
: {{object | filter}}. Additional information for each of the filters is listed below:
Filter = at_least
Limits a number to a minimum value.
INPUT | OUTPUT |
---|---|
| 5 |
| 4 |
Filter = currency
Use the following Liquid filter for Currency Formatting:
CODE
|
Amount | Code |
---|---|
$1,000.50 |
CODE
|
Below is a list of country/language combinations:
County/Language Combinations |
---|
|
Filter = abs
Returns the absolute value of a number:
INPUT | OUTPUT |
---|---|
| 17 |
INPUT | OUTPUT |
---|---|
| 4 |
abs
will also work on a string that only contains a number:
INPUT | OUTPUT |
---|---|
| 19.86 |
Filter = append
INPUT | OUTPUT |
---|---|
| /my/fancy/url.html |
append
can also be used with variables:
INPUT | OUTPUT |
---|---|
|
Filter = at_most
Limits a number to a maximum value.
INPUT | OUTPUT |
---|---|
| 4 |
INPUT | OUTPUT |
---|---|
| 3 |
Filter = capitalize
Makes the first character of a string capitalized.
INPUT | OUTPUT |
---|---|
| Title |
Capitalize
only capitalizes the first character of a string, so later words are not affected:
INPUT | OUTPUT |
---|---|
| My great title |
Filter = ceil
Rounds the input up to the nearest whole number. Liquid tries to convert the input to a number before the filter is applied:
INPUT | OUTPUT |
---|---|
| 2 |
INPUT | OUTPUT |
---|---|
| 2 |
INPUT | OUTPUT |
---|---|
| 184 |
Here the input value is a string:
INPUT | OUTPUT |
---|---|
| 4 |
Filter= compact
Removes any nil
values from an array.
For this example, assume site.pages
is an array of content pages for a website, and some of these pages have an attribute called category
that specifies their content category. If we map
those categories to an array, some of the array items might be nil
if any pages do not have a category
attribute.
INPUT | OUTPUT |
---|---|
|
|
By using compact
when we create our site_categories
array, we can remove all the nil
values in the array.
INPUT | OUTPUT |
---|---|
|
|
Filter= concat
Concatenates (joins together) multiple arrays. The resulting array contains all the items from the input arrays:
INPUT | OUTPUT |
---|---|
|
|
You can string together concat
filters to join more than two arrays:
INPUT | OUTPUT |
---|---|
|
|
Filter = date
Converts a timestamp into another date format. The format for this syntax is the same as strftime
. The input uses the same format as Ruby’s Time.parse
.
INPUT | OUTPUT |
---|---|
| Fri, Jul 17, 15 |
INPUT | OUTPUT |
---|---|
| 2015 |
date
works on strings if they contain well-formatted dates:
INPUT | OUTPUT |
---|---|
| Mar 14, 16 |
To get the current time, pass the special word "now" (or "today") to date:
INPUT | OUTPUT |
---|---|
| This page was last updated at 2019-09-19 17:48. |
The value will be the current time of when the page was last generated from the template, not when the page is presented to a user if caching or static site generation is involved.
Filter = default
Allows you to specify a fallback in case a value doesn’t exist. default
will show its value if the left side is nil
, false
, or empty. In this example, product_price
is not defined, so the default value is used.
INPUT | OUTPUT |
---|---|
| 2.99 |
In this example, product_price
is defined, so the default value is not used.
INPUT | OUTPUT |
---|---|
| 4.99 |
In this example, product_price
is empty, so the default value is used.
INPUT | OUTPUT |
---|---|
| 2.99 |
Filter = divided_by
Divides a number by another number. The result is rounded down to the nearest integer (that is, the floor) if the divisor is an integer.
INPUT | OUTPUT |
---|---|
| 4 |
INPUT | OUTPUT |
---|---|
| 1 |
Controlling Rounding
divided_by
produces a result of the same type as the divisor — that is, if you divide by an integer, the result will be an integer. If you divide by a float (a number with a decimal in it), the result will be a float. For example, here the divisor is an integer:
INPUT | OUTPUT |
---|---|
| 2 |
Here it is a float:
INPUT | OUTPUT |
---|---|
| 2.857142857142857 |
Changing Variable Types
You might want to use a variable as a divisor, in which case you can’t simply add .0
to convert it to a float. In these cases, you can assign
a version of your variable converted to a float using the times
filter. In this example, we’re dividing by a variable that contains an integer, so we get an integer:
INPUT | OUTPUT |
---|---|
| 2 |
Here, we multiply the variable by 1.0
to get afloat, then divide by the float instead:
INPUT | OUTPUT |
---|---|
| 2.857142857142857 |
Filter = downcase
Makes each character in a string lowercase. It has no effect on strings that are already all lowercase.
INPUT | OUTPUT |
---|---|
| parker moore |
INPUT | OUTPUT |
---|---|
| apple |
Filter = escape
Escapes a string by replacing characters with escape sequences (so that the string can be used in a URL, for example). It doesn’t change strings that don’t have anything to escape.
INPUT | OUTPUT |
---|---|
| Have you read 'James & the Giant Peach'? |
INPUT | OUTPUT |
---|---|
| Tetsuro Takara |
Filter = escape_once
Escapes a string without changing existing escaped entities. It doesn’t change strings that don’t have anything to escape.
INPUT | OUTPUT |
---|---|
| 1 < 2 & 3 |
INPUT | OUTPUT |
---|---|
| 1 < 2 & 3 |
Filter = first
Returns the first item of an array.
INPUT | OUTPUT |
---|---|
| Ground |
INPUT | OUTPUT |
---|---|
| zebra |
You can use "first"
with dot notation when you need to use the filter inside a tag:
Example |
---|
|
Filter = floor
Rounds the input down to the nearest whole number. Liquid tries to convert the input to a number before the filter is applied.
INPUT | OUTPUT |
---|---|
| 1 |
INPUT | OUTPUT |
---|---|
| 2 |
INPUT | OUTPUT |
---|---|
| 183 |
Here the input value is a string:
INPUT | OUTPUT |
---|---|
| 3 |
Filter = join
Combines the items in an array into a single string using the argument as a separator.
INPUT | OUTPUT |
---|---|
| John and Paul and George and Ringo |
Filters = last
Returns the last item of an array.
INPUT | OUTPUT |
---|---|
| Tom |
INPUT | OUTPUT |
---|---|
| tiger |
You can use "last"
with dot notation when you need to use the filter inside a tag:
Example |
---|
|
Filter = lstrip
Removes all whitespace (tabs, spaces, and newlines) from the left side of a string. It does not affect spaces between words.
INPUT | OUTPUT |
---|---|
| So much room for activities! |
Filters = map
It creates an array of values by extracting the values of a named property from another object. In this example, assume the object site.pages
contain all the metadata for a website. Using assign
with the map
filter creates a variable that contains only the values of the category
properties of everything on the site.pages
object.
INPUT | OUTPUT |
---|---|
|
|
Filter= minus
Subtracts a number from another number.
INPUT | OUTPUT |
---|---|
| 2 |
INPUT | OUTPUT |
---|---|
| 12 |
INPUT | OUTPUT |
---|---|
| 171.357 |
Filter = modulo
Returns the remainder of a division operation.
INPUT | OUTPUT |
---|---|
| 1 |
INPUT | OUTPUT |
---|---|
| 3 |
INPUT | OUTPUT |
---|---|
| 3.357 |
Filter = newline_to_br
Replaces every newline (\n
) in a string with an HTML line break (<br />
).
INPUT | OUTPUT |
---|---|
| <br /> Hello<br /> there<br /> |
Filter = plus
Adds a number to another number.
INPUT | OUTPUT |
---|---|
| 6 |
INPUT | OUTPUT |
---|---|
| 20 |
INPUT | OUTPUT |
---|---|
| 195.357 |
Filter= prepend
Adds the specified string to the beginning of another string.
INPUT | OUTPUT |
---|---|
| Some fruit: apples, oranges, and bananas |
"prepend"
can also be used with variables:
INPUT | OUTPUT |
---|---|
|
Filter = remove
Removes every occurrence of the specified substring from a string.
INPUT | OUTPUT |
---|---|
| I sted to see the t through the |
Filter = remove_first
Removes only the first occurrence of the specified substring from a string.
INPUT | OUTPUT |
---|---|
| I sted to see the train through the rain |
Filter = replace
Replaces every occurrence of the first argument is a string with the second argument.
INPUT | OUTPUT |
---|---|
| Take your protein pills and put your helmet on |
Filter = replace_first
Replaces only the first occurrence of the first argument is a string with the second argument.
INPUT | OUTPUT |
---|---|
| Take your protein pills and put my helmet on |
Filter = reverse
Reverses the order of the items in an array. reverse
cannot reverse a string.
INPUT | OUTPUT |
---|---|
| plums, peaches, oranges, apples |
Although reverse
cannot be used directly on a string, you can split a string into an array, reverse the array, and rejoin it by chaining together filters:
INPUT | OUTPUT |
---|---|
| .moT rojaM ot lortnoc dnuorG |
Filter = round
Rounds a number to the nearest integer or, if a number is passed as an argument, to that number of decimal places.
INPUT | OUTPUT |
---|---|
| 1 |
INPUT | OUTPUT |
---|---|
| 3 |
INPUT | OUTPUT |
---|---|
| 183.36 |
Filter= rstrip
Removes all whitespace (tabs, spaces, and newlines) from the right side of a string. It does not affect spaces between words.
INPUT | OUTPUT |
---|---|
| So much room for activities! |
Filter = size
Returns the number of characters in a string or the number of items in an array.
INPUT | OUTPUT |
---|---|
| 28 |
INPUT | OUTPUT |
---|---|
| 4 |
You can use "size"
with dot notation when you need to use the filter inside a tag:
Example |
---|
|
Filter = slice
Returns a substring of 1 character beginning at the index specified by the first argument. An optional second argument specifies the length of the substring to be returned.
String indices are numbered starting from 0.
INPUT | OUTPUT |
---|---|
| L |
INPUT | OUTPUT |
---|---|
| q |
INPUT | OUTPUT |
---|---|
| quid |
If the first argument is a negative number, the indices are counted from the end of the string:
INPUT | OUTPUT |
---|---|
| ui |
Filter = sort
Sorts items in an array in case-sensitive order.
INPUT | OUTPUT |
---|---|
| Sally Snake, giraffe, octopus, zebra |
An optional argument specifies which property of the array’s items to use for sorting.
Example |
---|
|
Filter = sort_natural
Sorts items in an array in case-sensitive order.
INPUT | OUTPUT |
---|---|
| Sally Snake, giraffe, octopus, zebra |
An optional argument specifies which property of the array’s items to use for sorting.
Example |
---|
|
Filter = split
Divides a string into an array using the argument as a separator. "split"
is commonly used to convert comma-separated items from a string to an array.
INPUT | OUTPUT |
---|---|
|
|
Filter = strip
Removes all whitespace (tabs, spaces, and newlines) from both the left and right sides of a string. It does not affect spaces between words.
INPUT | OUTPUT |
---|---|
| So much room for activities! |
Filter = strip_html
Removes any HTML tags from a string.
INPUT | OUTPUT |
---|---|
| Have you read Ulysses? |
Filter = strip_newlines
Removes any newline characters (line breaks) from a string.
INPUT | OUTPUT |
---|---|
| Hellothere |
Filter = times
Multiplies a number by another number.
INPUT | OUTPUT |
---|---|
| 6 |
INPUT | OUTPUT |
---|---|
| 168 |
INPUT | OUTPUT |
---|---|
| 2200.284 |
Filter = title_case
Converts strings to title case.
INPUT | OUTPUT |
---|---|
| My Great Title |
Filter = truncate
Shortens a string down to the number of characters passed as an argument. If the specified number of characters is less than the length of the string, an ellipsis (…) is appended to the string and is included in the character count.
INPUT | OUTPUT |
---|---|
| Ground control to... |
Custom Ellipsis
"truncate"
takes an optional second argument that specifies the sequence of characters to be appended to the truncated string. By default, this is an ellipsis (…), but you can specify a different sequence.
The length of the second argument counts against the number of characters specified by the first argument. For example, if you want to truncate a string to exactly 10 characters, and use a 3-character ellipsis, use 13 for the first argument of truncate
, since the ellipsis counts as 3 characters.
INPUT | OUTPUT |
---|---|
| Ground control, and so on |
No Ellipsis
You can truncate to the exact number of characters specified by the first argument and avoid showing trailing characters by passing a blank string as the second argument:
INPUT | OUTPUT |
---|---|
| Ground control to Ma |
Filter = truncatewords
Shortens a string down to the number of words passed as an argument. If the specified number of words is less than the number of words in the string, an ellipsis (…) is appended to the string.
INPUT | OUTPUT |
---|---|
| Ground control to... |
Custom Ellipsis
"truncatewords"
takes an optional second argument that specifies the sequence of characters to be appended to the truncated string. By default, this is an ellipsis (…), but you can specify a different sequence.
INPUT | OUTPUT |
---|---|
| Ground control to-- |
No Ellipsis
You can avoid showing trailing characters by passing a blank string as the second argument:
INPUT | OUTPUT |
---|---|
| Ground control to |
Filter = uniq
Removes any duplicate elements in an array.
INPUT | OUTPUT |
---|---|
| ants, bugs, bees |
Filter = upcase
Makes each character in a string uppercase. It has no effect on strings that are already all uppercase.
INPUT | OUTPUT |
---|---|
| PARKER MOORE |
INPUT | OUTPUT |
---|---|
| APPLE |
Filters = url_decode
Decodes a string that has been encoded as a URL or by url_encode.
INPUT | OUTPUT |
---|---|
| 'Stop!' said Fred |
Filter = url_encode
Converts any URL-unsafe characters in a string into percent-encoded characters.
INPUT | OUTPUT |
---|---|
| john%40liquid.com |
INPUT | OUTPUT |
---|---|
| Tetsuro+Takara |
Filter = where
Creates an array including only the objects with a given property value, or any truthy value by default. In this example, assume you have a list of products and you want to show your kitchen products separately. Using where
, you can create an array containing only the products that have a type of kitchen.
INPUT | OUTPUT |
---|---|
All products: | All Products:
Kitchen Products:
|
Say instead you have a list of products and you only want to show those that are available to buy. You can use where
with a property name but no target value to include all products with a truthy "available" value.
INPUT | OUTPUT |
---|---|
All products:
Available products:
| All Products:
Available Products:
|
The where
filter can also be used to find a single object in an array when combined with the "first" filter. For example, say you want to show off the shirt in your new fall collection.
INPUT | OUTPUT |
---|---|
| Featured Product: Hawaiian print sweater vest |
Filter = encrypt or decrypt
The Zeta Marketing Platform offers two types of encryption and decryption methods, AES and DES.
AES
encrypt |
---|
|
decrypt |
---|
|
DES
encrypt |
---|
|
decrypt |
---|
|
Filter = sha256
Creates a SHA256 hash of the data. This is a one-way function and cannot be reversed.
Example |
---|
|
Filter = base64_encode
Encodes data as a base64 string. Can be decoded using the base64_decode
filter.
Example |
---|
|
Filter = base64_decode
Used to decode base64 encoded data.
Example Usage |
---|
|